Curve
A curve is a type of Evaluator representing a mathematical function transforming one value into another one. Grail’s curves operate on floating point numbers (R→R).
Each curve has to be informed which Context may be passed through it during evaluation. Contexts can take form of any built-in or user-defined data type.
A user can inherit from Curve
class to create their own custom curves or they can make use of Grail’s built-in curve types.
Basic Curves
Let’s assume that x
means input value.
Step Function
-
threshold
-
beforeThresholdValue
-
afterThresholdValue
This function returns afterThresholdValue
if x
is greater than threshold
.
Otherwise returns beforeThresholdValue
.
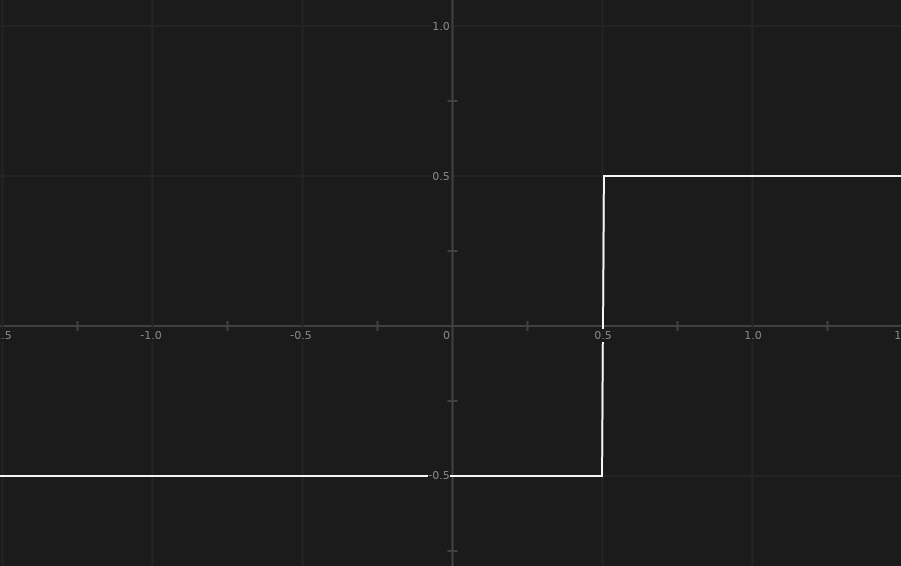
Linear Function
-
slope
-
intercept
This functions returns result of operation slope
* x
+ intercept
.
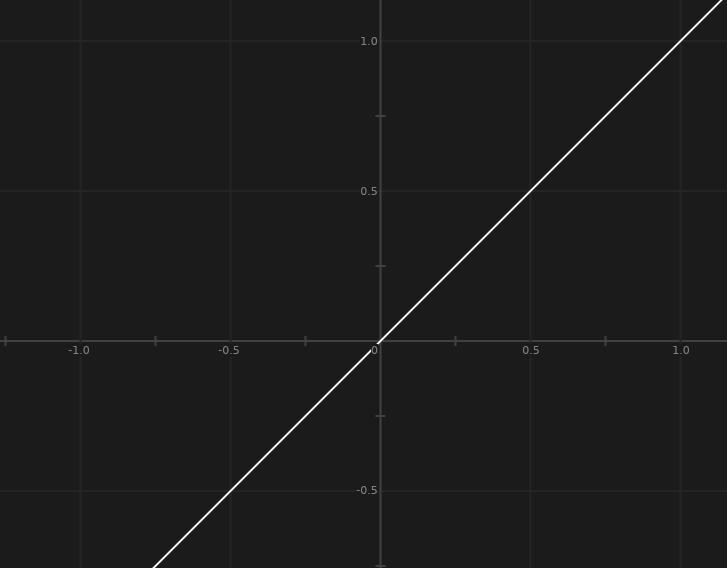
Power Function
-
exponent
-
slope
-
intercept
This function takes linear function and raises it to power determined by exponent
The result of linear function slope
* x
+ intercept
is lowerbound by 0
.
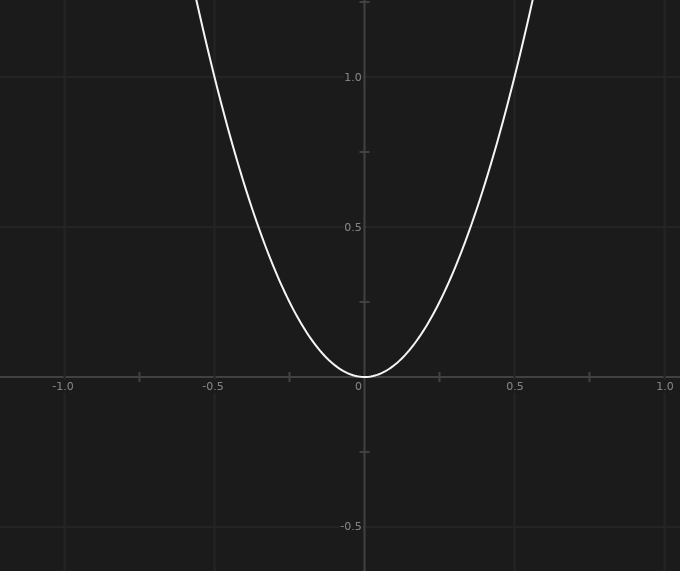
Sigmoid Function
-
slope
-
threshold
Work in progress. One day it will become a properly working sigmoid function.
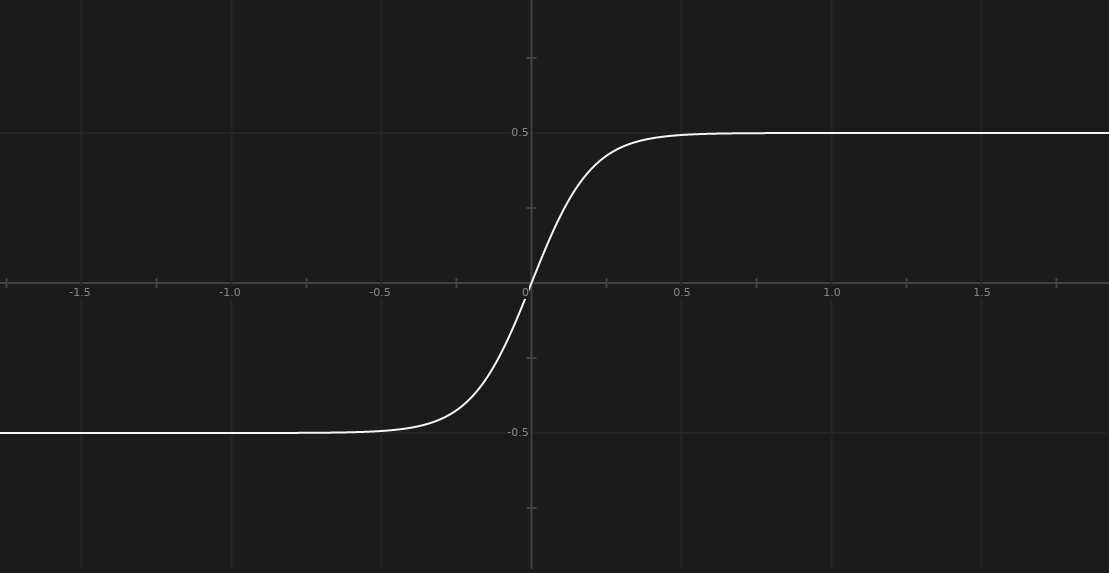
Bounded Curves
Lowerbound
Lowerbound curves cannot return result lesser than their lowerValue
.
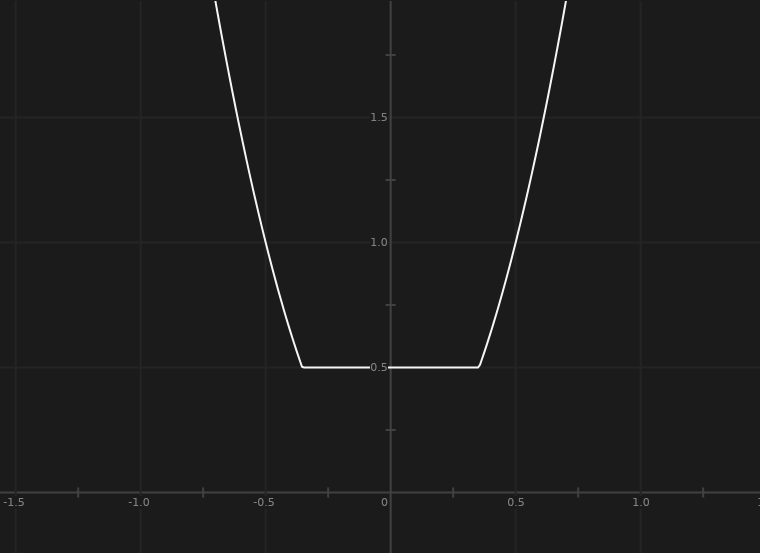
auto linearCurve = std::make_shared<LinearFunction<ContextType>(someConsideration, 1, 0);
auto lowerbound = std::make_shared<LowerBound<ContextType>>(linearCurve, 0); // linearCurve bounded to range <0;inf>
var linearCurve = new LinearFunction<ContextType>(someConsideration, 1, 0);
var lowerbound = new LowerBound<ContextType>(linearCurve, 0); // linearCurve bounded to range <0;inf>
Upperbound
Upperbound curves cannot return result greater than their upperValue
.
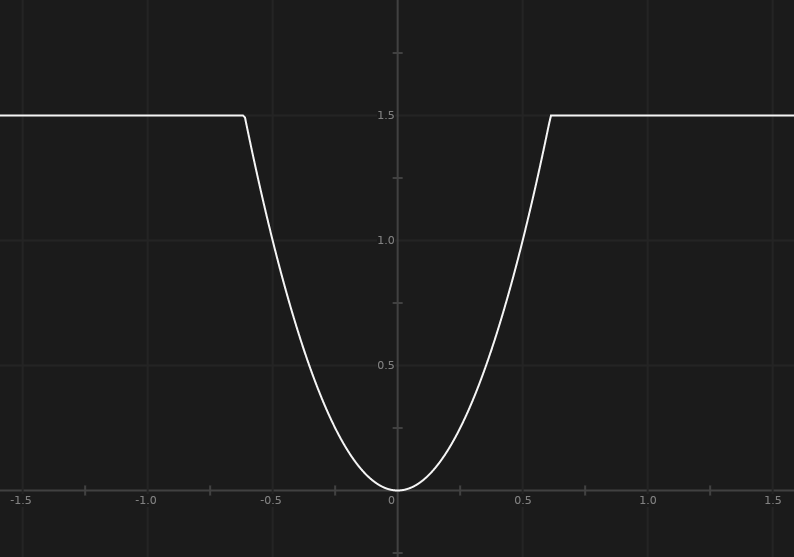
auto someConsideration = std::make_shared<SomeConsideration>();
auto upperbound = std::make_shared<UpperBound<ContextType>>(someConsideration, 1); // someConsideration bounded to range <-inf;1>
var someConsideration = new SomeConsideration();
var upperbound = new UpperBound(someConsideration, 1); // someConsideration bounded to range <-inf;1>