Curve
A curve is a mathematical function transforming one value into another one. Grail’s curves operate on floating point numbers (R→R). They are often used in Grail’s utility evaluators.
A user can inherit from Curve
class to create their own custom curves or they can make use of Grail’s built-in curve types.
Basic Curves
Let’s assume that x
means input value.
Step Function
-
threshold
-
beforeThresholdValue
-
afterThresholdValue
This function returns afterThresholdValue
if x
is greater than threshold
.
Otherwise returns beforeThresholdValue
.
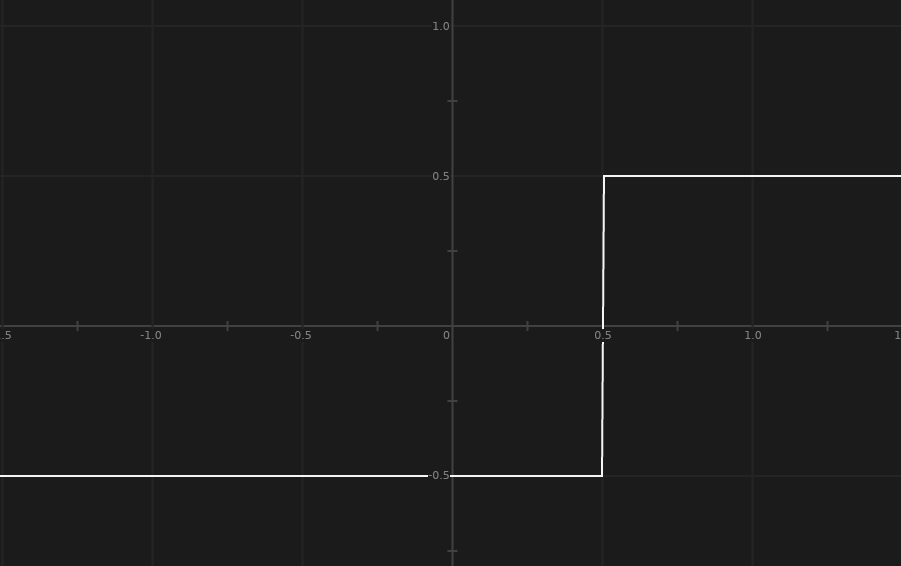
Linear Function
-
slope
-
intercept
This functions returns result of operation slope
* x
+ intercept
.
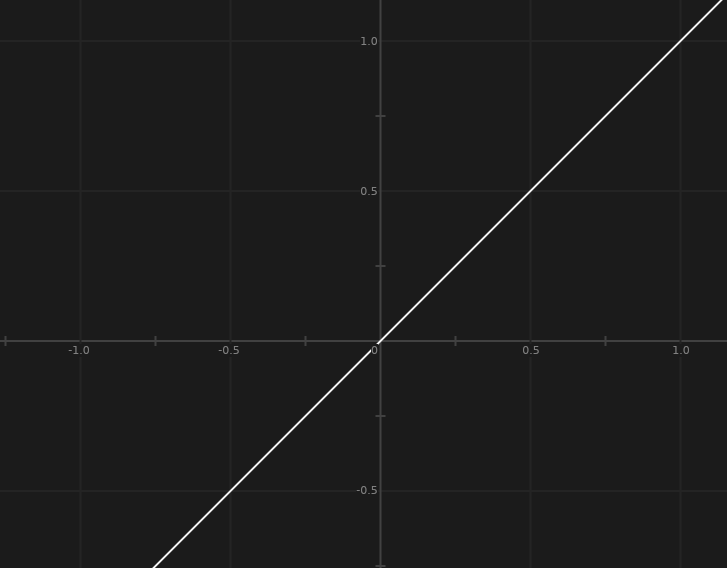
Power Function
-
exponent
-
slope
-
intercept
This function takes linear function and raises it to power determined by exponent
The result of linear function slope
* x
+ intercept
is lowerbound by 0
.
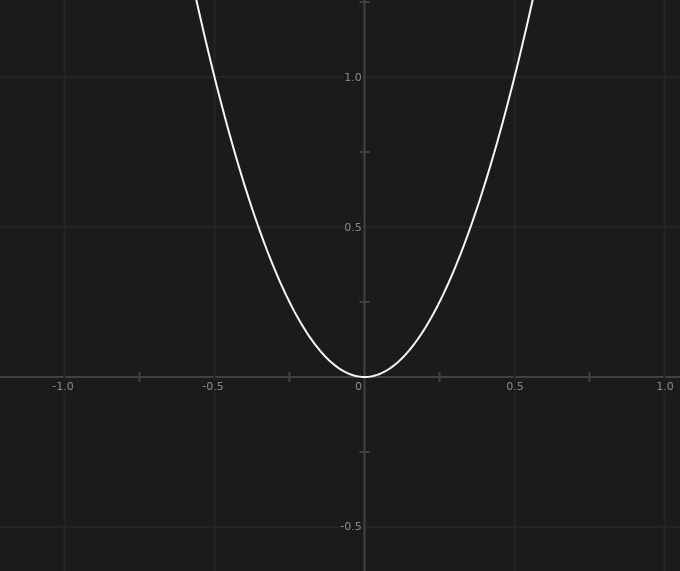
Sigmoid Function
-
slope
-
threshold
Work in progress. One day it will become a properly working sigmoid function.
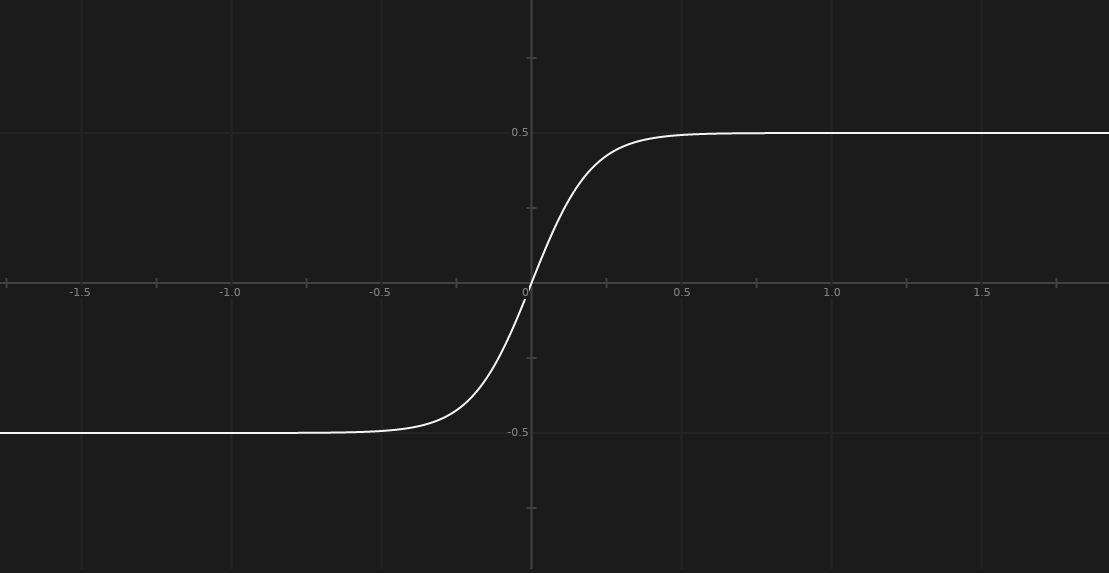