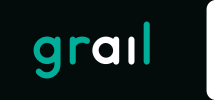 |
Grail (C++)
1.4.0
A multi-platform, modular, universal engine for embedding advanced AI in games.
|
3 #ifndef GRAIL_PLANNER_H
4 #define GRAIL_PLANNER_H
6 #include "../../GrailData/DebugInfo/PlannerSnapshots.h"
7 #include "Action/Action.hh"
8 #include "WorldState/WorldState.hh"
26 using ConditionFunction = std::function<bool(
const WorldState&)>;
32 using PlannerHeuristic = std::function<double(
const WorldState&,
const std::vector<ConditionFunction>&)>;
64 std::size_t initialNodeCapacity = 128;
72 std::vector<Action> actions{};
73 float totalCost = 0.0f;
141 void Iterate(std::size_t iterationCount) noexcept;
153 std::size_t minDebugSnapshotIteration = 0,
154 std::size_t maxDebugSnapshotIteration = std::numeric_limits<std::size_t>::max())
192 double distanceFromGoal = std::numeric_limits<double>::max();
193 std::shared_ptr<Edge> pathEdge =
nullptr;
195 Node(Node&& other) noexcept;
198 Node& operator=(Node&& other) noexcept;
203 Edge(std::size_t sourceIndex, std::size_t destinationIndex,
Action&& action);
204 Edge(Edge&& otherEdge) =
default;
206 std::size_t sourceIndex = 0;
207 std::size_t destinationIndex = 0;
213 EdgePriority(
const std::shared_ptr<Edge>& edge,
double dijkstraScore,
double aStarScore);
215 std::shared_ptr<Edge> edge;
216 double dijkstraScore;
220 class EdgePriorityComparer
223 bool operator()(
const EdgePriority& one,
const EdgePriority& other)
225 return one.aStarScore > other.aStarScore;
231 void AddNode(Node&& node);
232 bool IsGoalAchieved(
const WorldState& state)
const;
234 std::unique_ptr<WorldState> initialState;
235 Config plannerConfig = {};
237 std::vector<ConditionFunction> goalConditions = {};
238 std::function<void(
const AbstractPlan&)> onPlanFound =
nullptr;
239 std::function<void()> onPlanningFailed =
nullptr;
242 std::size_t nextNodeIndex = 0;
243 std::size_t nodeClosestToGoalIndex = std::numeric_limits<std::size_t>::max();
244 std::size_t lastChosenNodeIndex = std::numeric_limits<std::size_t>::max();
245 int maxNodeDepth = 0;
246 std::priority_queue<EdgePriority, std::vector<EdgePriority>, EdgePriorityComparer> edges{};
247 std::vector<Node> generatedNodes{};
248 std::vector<std::size_t> closedNodeIndices{};
249 std::size_t currentIteration = 0;
250 bool isPlanningFinished =
true;
254 #endif //GRAIL_PLANNER_H
void SetHeuristic(PlannerHeuristic heuristic)
Definition: Planner.cpp:49
void SetPlanFoundCallback(const std::function< void(const AbstractPlan &)> callbackFunction)
SetPlanFoundCallback - Binds a function handle to call if a sufficient plan is found....
Definition: Planner.cpp:381
bool IsComputing() const
IsComputing - Returns true if no suitable plan was found and planning hasn't failed....
Definition: Planner.cpp:405
void SetMaxPlanCost(double maxCost)
SetMaxPlanCost - Limits generated plans cost (measured as the sum of costs of actions it consists of)...
Definition: Planner.cpp:44
Represents a formulated plan as a sequence of actions and a cost.
Definition: Planner.hh:70
void SetConfig(const Config &config)
ResetConfig - Sets planner config: max iteration, cost, length, plan heuristic, and node count.
Definition: Planner.cpp:54
Definition: WorldState.hh:20
void PushCondition(ConditionFunction condition)
Definition: Planner.cpp:59
void SetMaxPlanLength(int maxLength)
SetMaxPlanLength - Limits generated plans to not exceed given number of steps to achieve goal conditi...
Definition: Planner.cpp:39
data::PlannerIterationSnapshot GetPlanSnapshot(std::size_t goalNodeIndex, std::size_t iteration) const
GetPlanSnapshot - Get debug data on a single iteration of a plan.
Definition: Planner.cpp:64
AbstractPlan GetPartialPlan() const
GetPartialPlan - returns currently best plan, regardless if goal conditions are met....
Definition: Planner.cpp:87
void IterateWithSnapshot(std::size_t iterationCount, data::PlannerReasonerSnapshot &snapshot, std::size_t minDebugSnapshotIteration=0, std::size_t maxDebugSnapshotIteration=std::numeric_limits< std::size_t >::max()) noexcept
IterateWithSnapshot - Formulates a plan trying to meet goalConditions from initialState....
Definition: Planner.cpp:105
Definition: PlannerSnapshots.h:68
Definition: Planner.hh:39
Definition: PlannerSnapshots.h:92
The Planner's run-time configuration parameters.
Definition: Planner.hh:43
PlannerHeuristic heuristic
Definition: Planner.hh:53
std::size_t iterationLimit
The maximum number of iterations per single planning instance.
Definition: Planner.hh:56
int maxPlanLength
The maximum number of actions (steps) a plan can take. Set -1 for unlimited plans.
Definition: Planner.hh:59
void Iterate(std::size_t iterationCount) noexcept
Formulate a plan trying to meet goalConditions from initialState. It will call "OnPlanningFailed" or ...
Definition: Planner.cpp:261
void SetIterationLimit(std::size_t iterationLimit)
SetIterationLimit - sets maximum number of iterations per each execution of the planning problem....
Definition: Planner.cpp:34
void Reset(std::unique_ptr< WorldState > initialState)
Reset - Resets planner to given world state. Any existing plans and goal conditions will be cleared.
Definition: Planner.cpp:391
void SetPlanningFailedCallback(const std::function< void()> callbackFunction)
SetPlanningFailedCallback - Binds a function handle to call if no sufficient plan was found.
Definition: Planner.cpp:386
double maxPlanCost
An upper bound on the plan cost measured as the sum of costs of actions it consists of....
Definition: Planner.hh:62