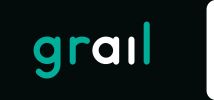 |
Grail (C++)
1.3.0
A multi-platform, modular, universal engine for embedding advanced AI in games.
|
3 #ifndef GRAIL_SIMULATED_GAMES_SNAPSHOTS_H
4 #define GRAIL_SIMULATED_GAMES_SNAPSHOTS_H
6 #include "Flatbuffers/SimulatedGameUnitSnapshot_generated.h"
7 #include "Flatbuffers/SimulatedGameActionMetadataSnapshot_generated.h"
8 #include "Flatbuffers/SimulatedGameMonteCarloEventSnapshot_generated.h"
9 #include "Flatbuffers/SimulatedGameUCTEventSnapshot_generated.h"
10 #include "Flatbuffers/SimulatedGameIterationSnapshot_generated.h"
11 #include "Flatbuffers/SimulatedGameReasonerSnapshot_generated.h"
15 #include <unordered_map>
22 class ISimulatedGameUnit;
41 std::map<std::string, std::string>
state = {};
47 flatbuffers::Offset<generated::SimulatedGameUnitSnapshot> Pack(flatbuffers::FlatBufferBuilder& builder,
48 const SimulatedGameUnitSnapshot& snapshot);
49 SimulatedGameUnitSnapshot Unpack(
const generated::SimulatedGameUnitSnapshot& snapshot);
68 flatbuffers::Offset<generated::SimulatedGameActionMetadataSnapshot> Pack(flatbuffers::FlatBufferBuilder& builder,
81 int activeUnitUID = 0;
87 flatbuffers::Offset<generated::SimulatedGameMonteCarloEventSnapshot> Pack(flatbuffers::FlatBufferBuilder& builder,
88 const SimulatedGameMonteCarloEventSnapshot& snapshot);
89 SimulatedGameMonteCarloEventSnapshot Unpack(
const generated::SimulatedGameMonteCarloEventSnapshot& snapshot);
95 std::vector<SimulatedGameActionMetadataSnapshot> actionMetadataSnapshots = {};
100 flatbuffers::Offset<generated::SimulatedGameUCTEventSnapshot> Pack(flatbuffers::FlatBufferBuilder& builder,
123 flatbuffers::Offset<generated::SimulatedGameIterationSnapshot> Pack(flatbuffers::FlatBufferBuilder& builder,
124 const SimulatedGameIterationSnapshot& snapshot);
125 SimulatedGameIterationSnapshot Unpack(
const generated::SimulatedGameIterationSnapshot& snapshot);
137 float absoluteTime = 0;
138 float relativeTime = 0;
142 flatbuffers::Offset<generated::SimulatedGameReasonerSnapshot> Pack(flatbuffers::FlatBufferBuilder& builder,
148 #endif //GRAIL_SIMULATED_GAMES_SNAPSHOTS_H
std::vector< SimulatedGameMonteCarloEventSnapshot > monteCarloSnapshots
Events that happened during the iteration in the Monte Carlo (simulation) phase.
Definition: SimulatedGamesSnapshots.h:114
Definition: SimulatedGamesSnapshots.h:74
std::vector< float > scores
Scores achieved by teams at the end of the iteration.
Definition: SimulatedGamesSnapshots.h:117
void Clear()
Clears all the stored data.
Definition: SimulatedGamesSnapshots.cpp:29
Definition: SimulatedGamesSnapshots.h:29
std::vector< SimulatedGameIterationSnapshot > iterationSnapshots
Snapshots of iterations contained in the SimulatedGameSnapshot.
Definition: SimulatedGamesSnapshots.h:135
std::string description
Display text for the unit in logs and GUI tool.
Definition: SimulatedGamesSnapshots.h:38
Definition: SimulatedGamesSnapshots.h:129
std::vector< SimulatedGameUnitSnapshot > unitSnapshots
States of units after action was performed.
Definition: SimulatedGamesSnapshots.h:80
int teamIndex
The "owning" player. Units of the same team should share the same color in the GUI tool.
Definition: SimulatedGamesSnapshots.h:32
Definition: SimulatedGamesSnapshots.h:93
std::map< std::string, std::string > state
State of the unit as serialized by FillDebugRepresentation method of a unit.
Definition: SimulatedGamesSnapshots.h:41
Base class of a unit in the SimulatedGame framework. A unit represents part of the game-state and pef...
Definition: ISimulatedGameUnit.hh:28
Definition: SimulatedGamesSnapshots.h:106
std::string chosenActionDescription
The action performed by the active unit.
Definition: SimulatedGamesSnapshots.h:77
std::vector< SimulatedGameUCTEventSnapshot > uctSnapshots
Events that happened during the iteration in the UCT (selection) phase.
Definition: SimulatedGamesSnapshots.h:111
int orderID
Ordered unique identifier of the unit. In this order they will apear in the GUI tool.
Definition: SimulatedGamesSnapshots.h:35
std::vector< SimulatedGameUnitSnapshot > unitSnapshots
Units that took part in the game.
Definition: SimulatedGamesSnapshots.h:132