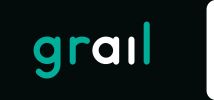 |
Grail (C++)
1.3.0
A multi-platform, modular, universal engine for embedding advanced AI in games.
|
3 #ifndef GRAIL_PLANNER_H
4 #define GRAIL_PLANNER_H
6 #include "../../GrailData/DebugInfo/PlannerSnapshots.h"
7 #include "Action/Action.hh"
8 #include "WorldState/WorldState.hh"
25 using ConditionFunction = std::function<bool(
const WorldState&)>;
26 using PlannerHeuristic = std::function<double(
const WorldState&,
const std::vector<ConditionFunction>&)>;
38 PlannerHeuristic heuristic =
nullptr;
39 std::size_t iterationLimit = 500;
40 int maxPlanLength = -1;
41 double maxPlanCost = -1;
42 std::size_t initialNodeCapacity = 128;
47 std::vector<Action> actions{};
48 float totalCost = 0.0f;
108 void Iterate(std::size_t iterationCount) noexcept;
120 std::size_t minDebugSnapshotIteration = 0,
121 std::size_t maxDebugSnapshotIteration = std::numeric_limits<std::size_t>::max())
159 double distanceFromGoal = std::numeric_limits<double>::max();
160 std::shared_ptr<Edge> pathEdge =
nullptr;
162 Node(Node&& other) noexcept;
165 Node& operator=(Node&& other) noexcept;
170 Edge(std::size_t sourceIndex, std::size_t destinationIndex,
Action&& action);
171 Edge(Edge&& otherEdge) =
default;
173 std::size_t sourceIndex = 0;
174 std::size_t destinationIndex = 0;
180 EdgePriority(
const std::shared_ptr<Edge>& edge,
double dijkstraScore,
double aStarScore);
182 std::shared_ptr<Edge> edge;
183 double dijkstraScore;
187 class EdgePriorityComparer
190 bool operator()(
const EdgePriority& one,
const EdgePriority& other)
192 return one.aStarScore > other.aStarScore;
198 void AddNode(Node&& node);
199 bool IsGoalAchieved(
const WorldState& state)
const;
201 std::unique_ptr<WorldState> initialState;
202 Config plannerConfig = {};
204 std::vector<ConditionFunction> goalConditions = {};
205 std::function<void(
const AbstractPlan&)> onPlanFound =
nullptr;
206 std::function<void()> onPlanningFailed =
nullptr;
209 std::size_t nextNodeIndex = 0;
210 std::size_t nodeClosestToGoalIndex = std::numeric_limits<std::size_t>::max();
211 std::size_t lastChosenNodeIndex = std::numeric_limits<std::size_t>::max();
212 int maxNodeDepth = 0;
213 std::priority_queue<EdgePriority, std::vector<EdgePriority>, EdgePriorityComparer> edges{};
214 std::vector<Node> generatedNodes{};
215 std::vector<std::size_t> closedNodeIndices{};
216 std::size_t currentIteration = 0;
217 bool isPlanningFinished =
true;
221 #endif //GRAIL_PLANNER_H
void SetHeuristic(PlannerHeuristic heuristic)
SetHeuristic - Gives planner a way to evaluate calculated plans.
Definition: Planner.cpp:49
void SetPlanFoundCallback(const std::function< void(const AbstractPlan &)> callbackFunction)
SetPlanFoundCallback - Binds a function handle to call if a sufficient plan is found....
Definition: Planner.cpp:381
bool IsComputing() const
IsComputing - Returns true if no suitable plan was found and planning hasn't failed....
Definition: Planner.cpp:405
void SetMaxPlanCost(double maxCost)
SetMaxPlanCost - Limits generated plans cost needed to achieve goal conditions.
Definition: Planner.cpp:44
Definition: Planner.hh:45
A class representing planner world state.
Definition: WorldState.hh:16
void PushCondition(ConditionFunction condition)
Definition: Planner.cpp:59
void SetMaxPlanLength(int maxLength)
SetMaxPlanLength - Limits generated plans to not exceed given number of steps to achieve goal conditi...
Definition: Planner.cpp:39
data::PlannerIterationSnapshot GetPlanSnapshot(std::size_t goalNodeIndex, std::size_t iteration) const
GetPlanSnapshot - Get debug data on a single iteration of a plan.
Definition: Planner.cpp:64
AbstractPlan GetPartialPlan() const
GetPartialPlan - returns currently best plan, regardless if goal conditions are met....
Definition: Planner.cpp:87
void IterateWithSnapshot(std::size_t iterationCount, data::PlannerReasonerSnapshot &snapshot, std::size_t minDebugSnapshotIteration=0, std::size_t maxDebugSnapshotIteration=std::numeric_limits< std::size_t >::max()) noexcept
IterateWithSnapshot - Formulates a plan trying to meet goalConditions from initialState....
Definition: Planner.cpp:105
Definition: PlannerSnapshots.h:68
The main class responsible for finding paths in plan space.
Definition: Planner.hh:29
void ResetConfig(const Config &config)
ResetConfig - Sets planner config: max iteration, cost, length, plan heuristic, and node count.
Definition: Planner.cpp:54
Definition: PlannerSnapshots.h:92
Definition: Planner.hh:32
void Iterate(std::size_t iterationCount) noexcept
Iterate - Formulate a plan trying to meet goalConditions from initialState. Evaluates plan using node...
Definition: Planner.cpp:261
void SetIterationLimit(std::size_t iterationLimit)
SetIterationLimit - sets maximum number of plans that can be generated during calculations.
Definition: Planner.cpp:34
void Reset(std::unique_ptr< WorldState > initialState)
Reset - Resets planner to given world state. Any existing plans and goal conditions will be cleared.
Definition: Planner.cpp:391
void SetPlanningFailedCallback(const std::function< void()> callbackFunction)
SetPlanningFailedCallback - Binds a function handle to call if no sufficient plan was found.
Definition: Planner.cpp:386