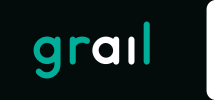 |
Grail (C++)
1.4.0
A multi-platform, modular, universal engine for embedding advanced AI in games.
|
3 #ifndef GRAIL_INDIVIDUAL_H
4 #define GRAIL_INDIVIDUAL_H
35 virtual std::unique_ptr<Individual>
Instantiate()
const = 0;
47 std::unique_ptr<Individual>
Clone()
const;
52 std::vector<std::unique_ptr<Individual>>
CloneMany(
int cloneCount)
const;
58 void Randomize(std::mt19937_64& randomGenerator);
68 std::mt19937_64& randomGenerator,
69 std::size_t count)
const;
81 virtual std::string
ToString()
const;
108 template<
typename ForwardIterator>
111 for (; beginIterator != endIterator; ++beginIterator)
118 std::vector<std::reference_wrapper<BaseEvoParam>>
parameters{};
121 void CopyEvoParamValuesFrom(
const Individual& other);
127 void ExchangeParams(
Individual& other, std::size_t inclusiveFrom, std::size_t exclusiveTo);
129 void SerializeState(std::ostream& outputStream)
const;
130 void DeserializeState(std::ifstream& inputStream);
133 double fitness = -1.0;
134 bool evaluationUpdated =
false;
138 std::vector<std::reference_wrapper<BaseEvoParam>>& GetEvoParams();
142 bool IndividualComparator(
const std::unique_ptr<Individual>& first,
const std::unique_ptr<Individual>& second);
145 #endif // GRAIL_INDIVIDUAL_H
BaseEvoParam - A class that represents a parameter that is modifiable (optimizable) by evolutionary a...
Definition: EvoParam.hh:20
bool Equals(const Individual &other) const
Tests whether two individuals have the same structure (even if they point to different objects in mem...
Definition: Individual.cpp:101
std::unique_ptr< Individual > Clone() const
Performs a deep copy of the individual.
Definition: Individual.cpp:27
float CalculateSimilarity(const Individual &other) const
CalculateSimilarity - this function calculates the similarity between two Individuals in the form of ...
Definition: Individual.cpp:129
The Crossover structure that encapsulates the crossover operation and its configuration in evolutiona...
Definition: Crossover.hh:40
void SetMemberParameterOptimizable(ForwardIterator beginIterator, ForwardIterator endIterator)
Call this method in a constructor and pass references to a field stored in this object....
Definition: Individual.hh:109
const BaseEvoParam & GetEvoParamAt(std::size_t index) const
GetEvoParamAt - returns the i-th EvoParam of the collection of parameters stored in this Individual.
Definition: Individual.cpp:91
std::vector< std::unique_ptr< Individual > > GetRandomRealizations(std::mt19937_64 &randomGenerator, std::size_t count) const
Returns "count" number of copies of the individuals with randomized parameter values.
Definition: Individual.cpp:66
double GetFitness() const
GetFitness - gets the fitness value (evaluation of how good the particular Individual object is).
Definition: Individual.cpp:22
std::size_t GetHashCode() const
Custom hash implementation.
Definition: Individual.cpp:96
void SetFitness(double fitness)
SetFitness - sets the fitness value (evaluation of how good the particular Individual object is).
Definition: Individual.cpp:16
long GetPossibleRealizationsCount() const
Definition: Individual.cpp:50
void Randomize(std::mt19937_64 &randomGenerator)
Changes the values of each parameter in the encoding of the individual to random available ones.
Definition: Individual.cpp:42
Represents an individual for evolutionary algorithms (EA). It stores the encoding consisting of opti...
Definition: Individual.hh:23
The Mutation structure that encapsulates the mutation operation and its configuration in evolutionary...
Definition: Mutation.hh:20
This class provides a functionality of assigning fitness values as averages from the previous evaluat...
Definition: FitnessRepository.h:24
virtual std::unique_ptr< Individual > Instantiate() const =0
Create your final optimizable object that is based on this Individual. In EA such object is often ref...
std::vector< std::unique_ptr< Individual > > CloneMany(int cloneCount) const
Performs a given number of copies of the individual.
Definition: Individual.cpp:35
std::size_t EvoParamsCount() const
EvoParamsCount - returns the number of parameters set as optimizable for evolutionary algorithms.
Definition: Individual.cpp:86
void SetMemberParameterOptimizable(BaseEvoParam ¶meter)
Call this method in a constructor and pass a reference to a field stored in this object....
Definition: Individual.cpp:202
std::vector< std::reference_wrapper< BaseEvoParam > > parameters
The encoding of the Individual – the collection of its optimizable parameters.
Definition: Individual.hh:118
virtual std::string ToString() const
ToString - returns the string representation of the Individual object.
Definition: Individual.cpp:155
A class that serves as the main interface for evolutionary algorithms in Grail.
Definition: EAOptimizer.hh:49