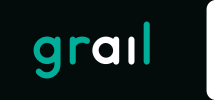 |
Grail (C++)
1.4.0
A multi-platform, modular, universal engine for embedding advanced AI in games.
|
6 #include "ActionTemplate.hh"
57 const std::string&
GetName()
const;
67 std::vector<unsigned int> argumentIndices{};
68 static thread_local std::vector<WorldObject*> effectFunctionArguments;
69 static thread_local std::vector<const WorldObject*> preconditionFunctionArguments;
74 #endif //GRAIL_ACTION_H
const std::vector< unsigned int > & GetArgumentIndices() const
Definition: Action.cpp:38
int GetType() const
Definition: Action.cpp:60
Definition: WorldState.hh:20
double GetCost(const WorldState &worldState) const
Definition: Action.cpp:28
Definition: ActionTemplate.hh:34
bool IsLegal(const WorldState &worldState) const
Definition: Action.cpp:18
void ApplyToState(WorldState &worldState) const
Definition: Action.cpp:43
Action(const ActionTemplate &actionTemplate, std::vector< unsigned int > &&argumentIndices)
Creates a new Action object based on the template.
Definition: Action.cpp:13
const std::string & GetName() const
Definition: Action.cpp:55