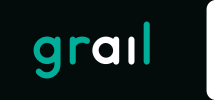 |
Grail (C++)
1.4.0
A multi-platform, modular, universal engine for embedding advanced AI in games.
|
3 #ifndef GRAIL_AI_MANAGER_H
4 #define GRAIL_AI_MANAGER_H
7 #include "EntityGroup.h"
8 #include "SynchronizedEntityContainer.h"
26 virtual void OnEntityRemoved(
const AIManager& manager,
size_t entityId) = 0;
62 void RegisterAIEntity(
const std::shared_ptr<AIEntity>& entity,
int priority);
124 const std::vector<std::pair<std::shared_ptr<AIEntity>,
int>>& GetEntities()
const;
125 const std::vector<std::shared_ptr<EntityGroup>>& GetEntityGroups()
const;
126 const std::map<std::string, std::shared_ptr<Blackboard>>& GetSharedBlackboards()
const;
130 void RequestProcessingEntities();
131 void ThreadUpdate(std::size_t
id);
133 void RemoveEntities();
134 void RegisterEntity(
const std::shared_ptr<AIEntity>& entity,
int priority);
136 std::vector<std::pair<std::shared_ptr<AIEntity>,
int>> entities{};
137 std::map<std::string, std::shared_ptr<Blackboard>> sharedBlackboards{};
140 std::atomic<bool> shouldTerminateWorkers = {0};
142 std::vector<std::thread> threads{};
143 std::mutex blackboardMutex{};
144 mutable std::mutex entitiesMutex{};
146 std::vector<std::shared_ptr<EntityGroup>> entityGroups{};
147 std::vector<IEntityChangeObserver*> entityChangeObservers{};
149 std::thread::id mainThreadId{};
152 #endif //GRAIL_AI_MANAGER_H
void RegisterAIEntity(const std::shared_ptr< AIEntity > &entity, int priority)
RegisterAIEntity - Manager registers an entity with a given priority. As a result entities and their ...
Definition: AIManager.cpp:141
void RegisterEntityGroup(const std::shared_ptr< EntityGroup > &entityGroup, int priority)
RegisterEntityGroup - Manager registers an entity group (and its entities) with the given priority....
Definition: AIManager.cpp:239
void RemoveAIEntity(AIEntity *entity)
RemoveAIEntity - Manager caches given entity. Later on all cached entities will be unregistered from ...
Definition: AIManager.cpp:206
The AIEntity class - Defines a basic object which can execute behaviors.
Definition: AIEntity.hh:50
The AIManager class - Manages registered entities and shared blackboards.
Definition: AIManager.hh:32
AIManager(std::size_t threadsNumber=0)
AIManager - Constructs a manager and spawns worker threads defined by the parameter....
Definition: AIManager.cpp:12
void UpdateEntities(float deltaTime)
UpdateEntities - Updates entities and their assigned behaviors.
Definition: AIManager.cpp:124
Blackboard & CreateBlackboard(const std::string &name)
CreateBlackboard - Constructs blackboard owned by this manager.
Definition: AIManager.cpp:308
bool ContainsEntity(const AIEntity &entity) const
ContainsEntity - Checks if a given entity is registered in the manager.
Definition: AIManager.cpp:271
The Blackboard class - grail's universal data container.
Definition: Blackboard.hh:15
void RemoveEntityChangeObserver(IEntityChangeObserver *observer)
RemoveEntityChangeObserver - Removes an entity change observer so it no longer will be notified on ad...
Definition: AIManager.cpp:348
void RegisterEntityChangeObserver(IEntityChangeObserver *observer)
RegisterEntityChangeObserver - Registers an entity change observer that will be notified via OnEntity...
Definition: AIManager.cpp:343
Definition: EntityToken.hh:8
Definition: AIManager.hh:15
void SubscribeToBlackboard(const std::string &blackboardName, AIEntity &entity)
SubscribeToBlackboard - Subscribes a given entity to a blackboard identified by the provided blackboa...
Definition: AIManager.cpp:316
void UpdateReasoners()
UpdateReasoners - Lets registered entities think by updating their reasoner.
Definition: AIManager.cpp:88
void RemoveEntityGroup(const std::shared_ptr< EntityGroup > &entityGroup)
RemoveEntityGroup - Manager removes entity group and caches its entities for removal....
Definition: AIManager.cpp:262
Definition: SynchronizedEntityContainer.h:15