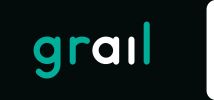 |
Grail (C++)
1.3.0
A multi-platform, modular, universal engine for embedding advanced AI in games.
|
3 #ifndef GRAIL_SINGLE_STEP_FUNCTION_H
4 #define GRAIL_SINGLE_STEP_FUNCTION_H
12 template <
typename ContextType>
29 float beforeThresholdValue,
30 float afterThresholdValue)
31 :
Curve<ContextType>{childEvaluator},
33 beforeThresholdValue{beforeThresholdValue},
34 afterThresholdValue{afterThresholdValue}
38 virtual float Sample(
float argument)
const override final
40 return argument > threshold ? afterThresholdValue : beforeThresholdValue;
59 virtual data::EvaluatorType GetEvaluatorType() const override final {
return data::EvaluatorType::CURVE_UNIT_STEP; }
62 float threshold = 0.0f;
63 float beforeThresholdValue = 0.0f;
64 float afterThresholdValue = 0.0f;
69 #endif // GRAIL_SINGLE_STEP_FUNCTION_H
UnitStepFunction(std::shared_ptr< Evaluator< ContextType >> childEvaluator, float threshold, float beforeThresholdValue, float afterThresholdValue)
UnitStepFunction - Constructor.
Definition: UnitStepFunction.hh:27
The Evaluator class - base class being able to evaluate given context and output the result.
Definition: Evaluator.hh:22
virtual float Sample(float argument) const override final
Sample - Transforms argument into output value depending on the type of Curve.
Definition: UnitStepFunction.hh:38
float GetAfterThresholdValue() const
GetAfterThresholdValue.
Definition: UnitStepFunction.hh:57
float GetThreshold() const
GetThreshold.
Definition: UnitStepFunction.hh:47
float GetBeforeThresholdValue() const
GetBeforeThresholdValue.
Definition: UnitStepFunction.hh:52
The UnitStepFunction class - Unit Step Function.
Definition: UnitStepFunction.hh:17
The Curve class - Defines objects transforming one value into the other.
Definition: Curve.hh:21