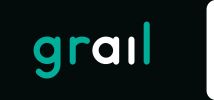 |
Grail (C++)
1.3.0
A multi-platform, modular, universal engine for embedding advanced AI in games.
|
3 #ifndef GRAIL_SUM_AGGREGATOR_H
4 #define GRAIL_SUM_AGGREGATOR_H
6 #include "Aggregator.hh"
12 template <
typename ContextType>
29 virtual data::EvaluatorType GetEvaluatorType() const override final {
return data::EvaluatorType::AGGREGATOR_SUM; }
36 for(
const auto& evaluator : this->childEvaluators)
38 weight += evaluator->EvaluateContext(context, snapshot);
SumAggregator(const std::vector< std::shared_ptr< Evaluator< ContextType >>> &childEvaluators)
SumAggregator - Constructor.
Definition: SumAggregator.hh:24
The Aggregator class - Base class for an Evaluator aggregating multiple other Evaluators' scores into...
Definition: Aggregator.hh:22
The Evaluator class - base class being able to evaluate given context and output the result.
Definition: Evaluator.hh:22
The SumAggregator class - Evaluator aggregating multiple other Evaluators' scores into one output by ...
Definition: SumAggregator.hh:17
The UtilityEvaluatorSnapshot class - debug snapshot of whole evaluator tree assigned to evaluated obj...
Definition: UtilityEvaluatorSnapshot.h:26
virtual float Evaluate(const ContextType &context, data::UtilityEvaluatorSnapshot *const snapshot) const override final
Evaluate - Called from EvaluateContext which also evaluates context, but without automatically fillin...
Definition: SumAggregator.hh:33