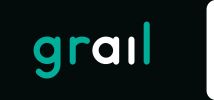 |
Grail (C++)
1.3.0
A multi-platform, modular, universal engine for embedding advanced AI in games.
|
3 #ifndef GRAIL_SIMULATED_GAME_SNAPSHOT_OBSERVER_H
4 #define GRAIL_SIMULATED_GAME_SNAPSHOT_OBSERVER_H
8 #include <unordered_map>
10 #include "SimulatedGameActionMetadata.hh"
11 #include "../GrailData/DebugInfo/SimulatedGamesSnapshots.h"
17 class SimulatedGameThinkingUnit;
18 class SimulatedGameStochasticUnit;
19 class ISimulatedGameAction;
20 class ISimulatedGameUnit;
21 class SimulatedGameNode;
34 void OnGameStart(
const std::vector<std::shared_ptr<SimulatedGameThinkingUnit>>& thinkingUnits,
35 const std::vector<std::shared_ptr<SimulatedGameStochasticUnit>>& stochasticUnits);
36 void OnIterationStart(
size_t iterationNumber);
39 void OnIterationEnd(
const std::vector<float>& scores);
58 std::unordered_map<const ISimulatedGameUnit*, int> idMap = {};
59 bool isInitialized =
false;
60 size_t minIteration = 0;
61 size_t maxIteration = std::numeric_limits<size_t>::max();
62 unsigned long globalIterationIndex = 0;
66 #endif //GRAIL_SIMULATED_GAME_SNAPSHOT_OBSERVER_H
Base class for all actions in SimulatedGame. Derive from it for your actions.
Definition: ISimulatedGameAction.hh:41
Definition: SimulatedGamesSnapshots.h:129
data::SimulatedGameReasonerSnapshot & GetSnapshot()
Returns serialized snapshot of SimulatedGameReasoner.
Definition: SimulatedGameSnapshotObserver.cpp:13
Definition: SimulatedGameSnapshotObserver.h:23
void SetMinIteration(size_t iteration)
Sets the minimum number of iteration that will be gathered during the debugging.
Definition: SimulatedGameSnapshotObserver.cpp:23
bool IsInitialized() const
Returns true if the observer is initialized and ready to gather data.
Definition: SimulatedGameSnapshotObserver.cpp:18
size_t GetMaxIteration() const
Gets the maximum number of iteration that will be gathered during the debugging.
Definition: SimulatedGameSnapshotObserver.cpp:38
void SetMaxIteration(size_t iteration)
Sets the maximum number of iteration that will be gathered during the debugging.
Definition: SimulatedGameSnapshotObserver.cpp:33
Base class of a unit in the SimulatedGame framework. A unit represents part of the game-state and pef...
Definition: ISimulatedGameUnit.hh:28
Definition: SimulatedGamesSnapshots.h:106
This class should not be visible to developers at all.
Definition: SimulatedGameNode.hh:25
size_t GetMinIteration() const
Gets the minimum number of iteration that will be gathered during the debugging.
Definition: SimulatedGameSnapshotObserver.cpp:28