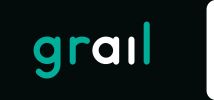 |
Grail (C++)
1.3.0
A multi-platform, modular, universal engine for embedding advanced AI in games.
|
3 #ifndef GRAIL_SIMULATED_GAME_REASONER_H
4 #define GRAIL_SIMULATED_GAME_REASONER_H
8 #include "../ISimulatedActionTranslator.hh"
9 #include "../ISimulatedGameStateTranslator.hh"
10 #include "../ISimulatedGameUnit.hh"
11 #include "../SimulatedGame.hh"
12 #include "../../GrailCore/AIEntity.hh"
13 #include "../../GrailCore/Plan.hh"
14 #include "../../GrailCore/Reasoner.hh"
20 struct SimulatedGameReasonerSnapshot;
25 class SimulatedGameSnapshotObserver;
39 std::unique_ptr<simgames::ISimulatedActionTranslator> actionTranslator,
40 std::size_t iterationsPerFrame,
41 std::size_t maxIterations = 1000,
42 std::size_t maxRecalculationIterations = 1000);
79 virtual std::unique_ptr<ISnapshotGenerator> CreateSnapshotGenerator(
size_t)
override;
86 std::unique_ptr<simgames::SimulatedGame> game;
87 std::unique_ptr<Behavior> fallbackBehavior;
89 std::unique_ptr<simgames::ISimulatedGameStateTranslator> worldStateTranslator;
90 std::unique_ptr<simgames::ISimulatedActionTranslator> actionTranslator;
93 std::size_t iterationsPerFrame{};
94 std::size_t maxIterations{};
95 std::size_t maxRecalculationIterations{};
96 std::size_t maxRecalculationIterationsLeft{};
97 std::size_t iterationCounter{};
99 const std::size_t minIterationsPerRun = 2;
101 std::shared_ptr<simgames::ISimulatedGameUnit> pairedAbstractUnit{};
102 std::unique_ptr<simgames::SimulatedGameSnapshotObserver> snapshotObserver{};
103 bool isGameDataReady =
false;
104 bool isComputing =
false;
105 bool isRecalculating =
false;
110 #endif // GRAIL_SIMULATED_GAME_REASONER_H
The AIEntity class - Defines a basic object which can execute behaviors.
Definition: AIEntity.hh:50
void SetDebugSnapshotFirstIteration(size_t iterationNumber)
You may limit the number of debug data collected to only data produced by simulations with numbers be...
Definition: SimulatedGameReasoner.cpp:139
SimulatedGameReasoner(std::unique_ptr< simgames::ISimulatedGameStateTranslator > worldStateTranslator, std::unique_ptr< simgames::ISimulatedActionTranslator > actionTranslator, std::size_t iterationsPerFrame, std::size_t maxIterations=1000, std::size_t maxRecalculationIterations=1000)
Constructs a new SimulatedGameReasoner
Definition: SimulatedGameReasoner.cpp:13
virtual void StageBehavior(AIEntity &entity) override
selectBehavior - Runs reasoner's selection algorithm and assigns chosen behavior to provided entity.
Definition: SimulatedGameReasoner.cpp:33
The Reasoner class - Entity's "brain", assigns them behaviors chosen by user-defined algorithms.
Definition: Reasoner.hh:21
Definition: SimulatedGameReasoner.hh:27
size_t GetDebugSnapshotFirstIteration() const
If the debug data is collected, this method will return the first iteration number from which the dat...
Definition: SimulatedGameReasoner.cpp:155
bool IsComputing() const
Returns true is the reasoner has not finished computations (i.e. there are still simulations to perfo...
Definition: SimulatedGameReasoner.cpp:165
The main interface class for the SimulatedGame reasoner based on the MCTS algorithm....
Definition: SimulatedGame.hh:30
void SetFallbackBehavior(std::unique_ptr< Behavior > _fallbackBehavior)
Set a fallback behavior if no valid behaviors are produced from actions returned by SimulatedGame....
Definition: SimulatedGameReasoner.cpp:89
const simgames::SimulatedGame & getGame() const
Gets the SimulatedGame object that is currently created by the ISimulatedGameStateTranslator.
Definition: SimulatedGameReasoner.cpp:94
Definition: SimulatedGamesSnapshots.h:129
A data structure used by PlannerReasoner to execute a sequence of behaviors.
Definition: Plan.hh:14
void RequestRecalculation(AIEntity &entity)
As documented, the ISimulatedActionTranslator translates the actions computed by SimulatedGame to beh...
Definition: SimulatedGameReasoner.cpp:99
void SetDebugSnapshotLastIteration(size_t iterationNumber)
You may limit the number of debug data collected to only data produced by simulations with numbers be...
Definition: SimulatedGameReasoner.cpp:147
void SetSnapshotProduction(bool shouldProduce)
Calling this function makes the reasoner collect debug data. The GrailDebugger will call it automatic...
Definition: SimulatedGameReasoner.cpp:127
size_t GetDebugSnapshotLastIteration() const
If the debug data is collected, this method will return the last iteration number up to which the dat...
Definition: SimulatedGameReasoner.cpp:160
data::SimulatedGameReasonerSnapshot ProduceDebugSnapshot()
This method returns debug snapshot of data collected so far by SimulatedGameSnapshotObserver....
Definition: SimulatedGameReasoner.cpp:175