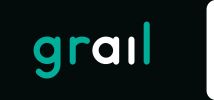 |
Grail (C++)
1.3.0
A multi-platform, modular, universal engine for embedding advanced AI in games.
|
3 #ifndef GRAIL_SIGMOID_FUNCTION_H
4 #define GRAIL_SIGMOID_FUNCTION_H
13 template <
typename ContextType>
34 :
Curve<ContextType>{childEvaluator},
38 displacement(displacement)
42 virtual float Sample(
float argument)
const override final
44 float x = slope * (argument - threshold);
47 float exp_x = std::exp(x);
48 return ((range * exp_x) / (1 + exp_x)) + displacement;
52 return (range / (1 + std::exp(-x))) + displacement;
77 virtual data::EvaluatorType GetEvaluatorType() const override final {
return data::EvaluatorType::CURVE_SIGMOID; }
82 float threshold = 0.0f;
83 float displacement = 0.0f;
88 #endif // GRAIL_SIGMOID_FUNCTION_H
virtual float Sample(float argument) const override final
Sample - Transforms argument into output value depending on the type of Curve.
Definition: SigmoidFunction.hh:42
float GetThreshold() const
GetThreshold.
Definition: SigmoidFunction.hh:70
The Evaluator class - base class being able to evaluate given context and output the result.
Definition: Evaluator.hh:22
float GetDisplacement() const
GetDisplacement.
Definition: SigmoidFunction.hh:75
The SigmoidFunction class - Sigmoid function.
Definition: SigmoidFunction.hh:18
float GetRange() const
GetRange.
Definition: SigmoidFunction.hh:60
SigmoidFunction(std::shared_ptr< Evaluator< ContextType >> childEvaluator, float range, float slope, float threshold, float displacement)
SigmoidFunction - Constructor.
Definition: SigmoidFunction.hh:29
The Curve class - Defines objects transforming one value into the other.
Definition: Curve.hh:21
float GetSlope() const
GetSlope.
Definition: SigmoidFunction.hh:65