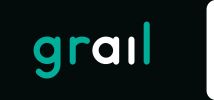 |
Grail (C++)
1.3.0
A multi-platform, modular, universal engine for embedding advanced AI in games.
|
3 #ifndef GRAIL_SELECTION_RESULT_H
4 #define GRAIL_SELECTION_RESULT_H
6 #include "../Blueprint.hh"
7 #include "../../GrailData/DebugInfo/UtilityReasonerSnapshot.h"
29 snapshot = std::make_unique<data::UtilityReasonerSnapshot>();
40 const std::string& evaluatedObjectMetadata,
45 snapshot->evaluatorSnapshots.emplace_back(evaluatedObjectName, evaluatedObjectMetadata, rank);
46 return &
snapshot->evaluatorSnapshots.back();
57 return optionIndex != std::numeric_limits<std::size_t>::max();
63 std::unique_ptr<data::UtilityReasonerSnapshot>
snapshot{
nullptr};
67 std::size_t
optionIndex = std::numeric_limits<std::size_t>::max();
72 #endif //GRAIL_SELECTION_RESULT_H
data::UtilityEvaluatorSnapshot * FetchNextEvaluatorSnapshot(const std::string &evaluatedObjectName, const std::string &evaluatedObjectMetadata, int rank)
FetchNextEvaluatorSnapshot - If debugging mode was enabled in constructor, creates an empty snapshot ...
Definition: SelectionResult.hh:39
std::size_t optionIndex
optionIndex - Index of option selected by Selector.
Definition: SelectionResult.hh:67
bool IsValid() const
IsValid - Checks whether Selector already finished it's calculations and encountered no errors.
Definition: SelectionResult.hh:55
The SelectionResult struct - Structure containing results of operations done by Selector.
Definition: SelectionResult.hh:19
The UtilityEvaluatorSnapshot class - debug snapshot of whole evaluator tree assigned to evaluated obj...
Definition: UtilityEvaluatorSnapshot.h:26
SelectionResult(bool isDebugging=false)
SelectionResult - Constructor.
Definition: SelectionResult.hh:25
std::unique_ptr< data::UtilityReasonerSnapshot > snapshot
snapshot - If debugging mode was enabled in constructor, contains debug snapshot of whole UtilityReas...
Definition: SelectionResult.hh:63