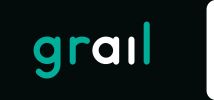 |
Grail (C++)
1.3.0
A multi-platform, modular, universal engine for embedding advanced AI in games.
|
3 #ifndef GRAIL_MUTATION_H
4 #define GRAIL_MUTATION_H
45 Mutation(
double mutationPhaseRate = 1.0,
46 double mutateIndividualRate = 0.09,
47 double mutateChromosomeRate = 0.5,
48 bool mutateBothParentsAndChildren =
true,
49 std::mt19937_64::result_type seed = std::random_device{}());
57 virtual void Perform(
const std::vector<std::unique_ptr<EvoScript>>& parentPopulation,
58 const std::vector<std::unique_ptr<EvoScript>>& childrenPopulation);
60 std::vector<std::unique_ptr<EvoScript>> populationAfter{};
77 std::mt19937_64 randGen;
82 virtual void MutateIndividual(
EvoScript& individual);
double MutateChromosomeRate
Probability that a chromosome of an individual will be considered for mutation (randomResolutionTypeF...
Definition: Mutation.hh:71
EvoParam - class that represents a parameter that is modifiable (optimizable) by evolutionary algorit...
Definition: EvoParam.hh:16
@ ITERATE_AND_TEST_P
ITERATE_AND_TEST_P -
MutationSelectionType
Predefined ways in which individuals are selected from the population for mutation....
Definition: Mutation.hh:26
EvoScript - a class that holds a collection of EvoParams (through them) is optimizable....
Definition: EvoScript.hh:18
bool MutateBothParentsAndChildren
If true: both the previous population and children can be mutated; if false: only the individuals aft...
Definition: Mutation.hh:74
@ SELECT_P_OF_N
SELECT_P_OF_N -
Mutation(double mutationPhaseRate=1.0, double mutateIndividualRate=0.09, double mutateChromosomeRate=0.5, bool mutateBothParentsAndChildren=true, std::mt19937_64::result_type seed=std::random_device{}())
Mutation - Constructor.
Definition: Mutation.cpp:10
The Mutation structure that encapsulates the mutation operation and its configuration in evolutionary...
Definition: Mutation.hh:20
double MutateIndividualRate
Probability that an individual will be considered for mutation (randomResolutionTypeForIndividual == ...
Definition: Mutation.hh:67
double MutationPhaseRate
Probability of a mutation phase to happen (globally). If a mutation is included in the algorithm,...
Definition: Mutation.hh:63
virtual void Perform(const std::vector< std::unique_ptr< EvoScript >> &parentPopulation, const std::vector< std::unique_ptr< EvoScript >> &childrenPopulation)
Perform - Attempts to mutate the entire population. The umber of individuals mutates is based on muta...
Definition: Mutation.cpp:27