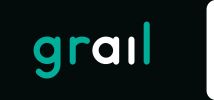 |
Grail (C++)
1.3.0
A multi-platform, modular, universal engine for embedding advanced AI in games.
|
3 #ifndef GRAIL_MAX_AGGREGATOR_H
4 #define GRAIL_MAX_AGGREGATOR_H
7 #include "Aggregator.hh"
13 template <
typename ContextType>
30 virtual data::EvaluatorType GetEvaluatorType() const override final {
return data::EvaluatorType::AGGREGATOR_MAX; }
36 float weight = std::numeric_limits<float>::min();
37 for(
const auto& evaluator : this->childEvaluators)
39 float current = evaluator->EvaluateContext(context, snapshot);
40 weight = std::max(weight, current);
The MaxAggregator class - Evaluator aggregating multiple other Evaluators' scores into one output by ...
Definition: MaxAggregator.hh:18
The Aggregator class - Base class for an Evaluator aggregating multiple other Evaluators' scores into...
Definition: Aggregator.hh:22
The Evaluator class - base class being able to evaluate given context and output the result.
Definition: Evaluator.hh:22
virtual float Evaluate(const ContextType &context, data::UtilityEvaluatorSnapshot *const snapshot) const override final
Evaluate - Called from EvaluateContext which also evaluates context, but without automatically fillin...
Definition: MaxAggregator.hh:34
MaxAggregator(const std::vector< std::shared_ptr< Evaluator< ContextType >>> &childEvaluators)
MaxAggregator - Constructor.
Definition: MaxAggregator.hh:25
The UtilityEvaluatorSnapshot class - debug snapshot of whole evaluator tree assigned to evaluated obj...
Definition: UtilityEvaluatorSnapshot.h:26