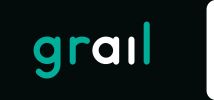 |
Grail (C++)
1.3.0
A multi-platform, modular, universal engine for embedding advanced AI in games.
|
3 #ifndef GRAIL_SIMULATED_GAME_ACTION_H
4 #define GRAIL_SIMULATED_GAME_ACTION_H
9 #include "SimulatedGameRuntime.hh"
18 template <
typename Po
interType>
19 std::size_t operator()(
const PointerType& p)
const
21 return p->HashForLearning();
28 template <
typename Po
interType>
29 bool operator()(
const PointerType& p1,
const PointerType& p2)
const
31 return p1->EqualsForLearning(*p2);
56 virtual std::string
ToString()
const;
74 #endif //GRAIL_SIMULATED_GAME_ACTION_H
virtual bool EqualsForLearning(const ISimulatedGameAction &other) const
Definition: ISimulatedGameAction.cpp:28
An interface that is used to terminate simulation in MCTS and set scores to players....
Definition: SimulatedGameRuntime.hh:17
virtual size_t HashForLearning() const
Definition: ISimulatedGameAction.cpp:23
Base class for all actions in SimulatedGame. Derive from it for your actions.
Definition: ISimulatedGameAction.hh:41
Helper that provides a HashForLearning() method. Used with ISimulatedGameAction only by OfflineLearni...
Definition: ISimulatedGameAction.hh:16
virtual ISimulatedGameUnit * Apply(ISimulatedGameUnit ¤tUnit, SimulatedGameRuntime &runtimeControl) const =0
Applies the effects of the action and returns the next Unit to make an action.
Helper that provides a EqualsForLearning() method. Used with ISimulatedGameAction only by OfflineLear...
Definition: ISimulatedGameAction.hh:26
Base class of a unit in the SimulatedGame framework. A unit represents part of the game-state and pef...
Definition: ISimulatedGameUnit.hh:28
virtual std::string ToString() const
Returns a text representation of an action - used only for printing and debugging purposes.
Definition: ISimulatedGameAction.cpp:13
virtual std::unique_ptr< ISimulatedGameAction > CloneForLearning() const
Definition: ISimulatedGameAction.cpp:18