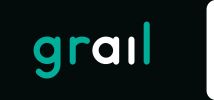 |
Grail (C++)
1.3.0
A multi-platform, modular, universal engine for embedding advanced AI in games.
|
3 #ifndef GRAIL_EXPONENTIAL_FUNCTION_H
4 #define GRAIL_EXPONENTIAL_FUNCTION_H
13 template <
typename ContextType>
27 :
Curve<ContextType>{childEvaluator}, base{base}
31 virtual float Sample(
float argument)
const override final
33 return std::pow(base, argument);
41 virtual data::EvaluatorType GetEvaluatorType() const override final {
return data::EvaluatorType::CURVE_EXPONENTIAL; }
49 #endif // GRAIL_EXPONENTIAL_FUNCTION_H
virtual float Sample(float argument) const override final
Sample - Transforms argument into output value depending on the type of Curve.
Definition: ExponentialFunction.hh:31
The Evaluator class - base class being able to evaluate given context and output the result.
Definition: Evaluator.hh:22
float GetBase() const
GetBase.
Definition: ExponentialFunction.hh:40
ExponentialFunction(std::shared_ptr< Evaluator< ContextType >> childEvaluator, float base)
ExponentialFunction - Construct.
Definition: ExponentialFunction.hh:26
The ExponentialFunction class - Exponential function.
Definition: ExponentialFunction.hh:18
The Curve class - Defines objects transforming one value into the other.
Definition: Curve.hh:21