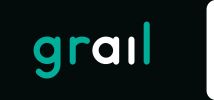 |
Grail (C++)
1.3.0
A multi-platform, modular, universal engine for embedding advanced AI in games.
|
3 #ifndef GRAIL_IEVO_PARAM_H
4 #define GRAIL_IEVO_PARAM_H
41 void Randomize(std::mt19937_64& rand_gen);
70 virtual std::string
ToString()
const;
74 #endif //GRAIL_IEVO_PARAM_H
EvoParam - class that represents a parameter that is modifiable (optimizable) by evolutionary algorit...
Definition: EvoParam.hh:16
const EvoParam * SetNext()
Sets to the next possible value in the domain (wrapped, i.e. last.next -> first) and returns itself.
Definition: EvoParam.cpp:14
const EvoParam * SetNextClamped()
Sets to the next possible value in the domain (clamped, i.e. last.next -> last) and returns itself.
Definition: EvoParam.cpp:26
const EvoParam * SetPrevClamped()
Sets to the previous possible value in the domain (clamped, i.e. first.prev -> first) and returns its...
Definition: EvoParam.cpp:32
EvoParam(size_t domainLength)
EvoParam - Constructor.
Definition: EvoParam.cpp:65
void Randomize(std::mt19937_64 &rand_gen)
Sets to a random possible value from a domain.
Definition: EvoParam.cpp:9
float GetNormalizedPositionWeight() const
Returns the normalized position as [0.0, 1.0] floating point number.
Definition: EvoParam.cpp:45
virtual std::string ToString() const
ToString - returns the string representation of the current value hold by the parameter.
Definition: EvoParam.cpp:60
const size_t domainLength
domainLength - the number of distinct values EvoParam may take.
Definition: EvoParam.hh:22
const EvoParam * SetPrev()
Sets to the previous possible value in the domain (wrapped, i.e. first.prev -> last) and returns itse...
Definition: EvoParam.cpp:20
size_t GetPositionIndex() const
Returns the position of the parameter i.e. the index of the currently assigned element from the domai...
Definition: EvoParam.cpp:50
size_t positionIndex
positionIndex - EvoParam uses positionIndex to point to an element from its domain.
Definition: EvoParam.hh:26
void SetPositionIndex(size_t position)
Sets the position of the parameter i.e. the index of the currently assigned element from the domain.
Definition: EvoParam.cpp:55