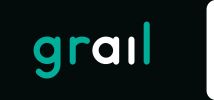 |
Grail (C++)
1.3.0
A multi-platform, modular, universal engine for embedding advanced AI in games.
|
3 #ifndef GRAIL_EVALUATION_DEBUG_DATA_H
4 #define GRAIL_EVALUATION_DEBUG_DATA_H
8 #include "../UtilityModel/EvaluatorType.h"
9 #include "Flatbuffers/EvaluationDebugData_generated.h"
13 template <
typename DataType>
73 void SetMetadata(
const std::string& newMetadata);
76 EvaluatorType evaluatorType{};
78 unsigned int nodeIndex{};
79 std::vector<unsigned int> childNodes{};
80 std::string metadata{};
83 flatbuffers::Offset<generated::EvaluationDebugData> Pack(flatbuffers::FlatBufferBuilder& builder,
const EvaluationDebugData& debugData);
The EvaluationDebugData class - debug data describing singular evaluator.
Definition: EvaluationDebugData.h:24
EvaluatorType GetEvaluatorType() const
GetEvaluatorType - provides type of the evalutor described by this debug data.
Definition: EvaluationDebugData.cpp:19
const std::string & GetMetadata() const
GetMetadata - provides a metadata string.
Definition: EvaluationDebugData.cpp:23
float GetScore() const
GetScore - provides output of the evaluator descibed by this debug data.
Definition: EvaluationDebugData.cpp:20
unsigned int GetNodeIndex() const
GetNodeIndex - provides this nodes position in debug tree.
Definition: EvaluationDebugData.cpp:21
void AddChildNode(unsigned int childNodeIndex)
AddChildNode - add node as leaf/child to this data node.
Definition: EvaluationDebugData.cpp:14
const std::vector< unsigned int > & GetChildNodes() const
GetChildNodes - provides indexes of all nodes attached to this node as children.
Definition: EvaluationDebugData.cpp:22