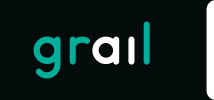 |
Grail (C++)
1.2.0
A multi-platform, modular, universal engine for embedding advanced AI in games.
|
3 #ifndef GRAIL_SIMULATED_GAME_RUNTIME_H
4 #define GRAIL_SIMULATED_GAME_RUNTIME_H
26 void SetScore(
int player,
float score);
37 std::vector<float> scores{};
39 bool terminationRequest =
false;
47 #endif //GRAIL_SIMULATED_GAME_RUNTIME_H
float GetScore(int player) const
Gets the score value set for a given player.
Definition: SimulatedGameRuntime.cpp:10
An interface that is used to terminate simulation in MCTS and set scores to players....
Definition: SimulatedGameRuntime.hh:17
void SetTerminationRequest()
Call this function to indicate that the game has ended, i.e. the terminal conditions have been met.
Definition: SimulatedGameRuntime.cpp:25
Definition: SimulatedGameHelper.h:18
The main interface class for the SimulatedGame reasoner based on the MCTS algorithm....
Definition: SimulatedGame.hh:30
void SetScore(int player, float score)
Sets value in the array of scores indexed by teams. See @teamCount in SimulatedGame constructor or @t...
Definition: SimulatedGameRuntime.cpp:15
bool IsTerminationRequest() const
Checks whether the terminationProperty has been set by calling the corresponding setTerminationReques...
Definition: SimulatedGameRuntime.cpp:20
This class should not be visible to developers at all.
Definition: SimulatedGameNode.h:25