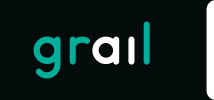 |
Grail (C++)
1.2.0
A multi-platform, modular, universal engine for embedding advanced AI in games.
|
3 #ifndef GRAIL_SIMULATED_GAME_HELPER_H
4 #define GRAIL_SIMULATED_GAME_HELPER_H
8 #include "SimulatedGameRuntime.hh"
14 class SimulatedGameNode;
15 class SimulatedGameSnapshotObserver;
21 void SetTeamCount(
int teamCount);
22 void SetExplorationRatio(
double explorationRatio);
23 void SetFreezeVisitsTreshold(
size_t treshold);
31 void Backpropagation();
36 double explorationConstant{};
37 size_t freezeVisitsTreshold{};
38 std::vector<SimulatedGameNode*> visitedNodes{};
39 std::vector<size_t> actionIndicesHistory{};
40 std::unique_ptr<SimulatedGameRuntime> runtimeControl{};
47 #endif //GRAIL_SIMULATED_GAME_HELPER_H
void ApplyActions() const
Applies deferred actions (to be called when the process falls out of the tree and Monte Carlo phase i...
Definition: SimulatedGameHelper.cpp:38
Definition: SimulatedGameHelper.h:18
Definition: SimulatedGameSnapshotObserver.h:23
void BackpropagationWithTerminal(std::vector< float > &scores)
This is backpropagation that checks whether a state is terminal.
Definition: SimulatedGameHelper.cpp:64
void Reset()
Resets to a state adequate to the start of a new simulation.
Definition: SimulatedGameHelper.cpp:31