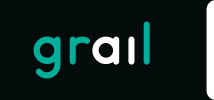 |
Grail (C++)
1.2.0
A multi-platform, modular, universal engine for embedding advanced AI in games.
|
3 #ifndef GRAIL_OFFLINE_LEARNER_H
4 #define GRAIL_OFFLINE_LEARNER_H
7 #include <unordered_map>
14 class ISimulatedGameUnit;
15 class SimulatedGameNode;
16 class SimulatedGameThinkingUnit;
21 class UniqueTreeDataset;
46 std::unique_ptr<UniqueTreeDataset>
GetSamplesDataset(
double minFractionOfSimulationsThreshold = 0.7);
58 double GetSampleMaxVisits()
const;
62 std::unique_ptr<IVectorizer> stateObserver;
63 std::unordered_map<const SimulatedGameNode*, std::vector<float>> capturedData = {};
71 #endif //GRAIL_OFFLINE_LEARNER_H
void ClearData()
Clears all data gathered for the game. You may want to call it after you already use the data but you...
Definition: OfflineLearner.cpp:20
void FillSamplesDataset(UniqueTreeDataset &dataset, double minFractionOfSimulationsThreshold=0.7)
Gets data gathered by OfflineLearner and adds it to dataset. Pass the container you want to add data ...
Definition: OfflineLearner.cpp:52
This is the main class you will use to peform the offline learning process. Assign it to the unit of ...
Definition: OfflineLearner.hh:27
A base class of a unit related to a rational/intelligent player. MCTS chooses actions for this kind o...
Definition: SimulatedGameThinkingUnit.hh:26
OfflineLearner(std::unique_ptr< IVectorizer > vectorizer)
Constructs a new OfflineLearner object.
Definition: OfflineLearner.cpp:15
std::unique_ptr< UniqueTreeDataset > GetSamplesDataset(double minFractionOfSimulationsThreshold=0.7)
Gets data gathered by OfflineLearner in the form of UniqueTreeDataset.
Definition: OfflineLearner.cpp:44
Base class of a unit in the SimulatedGame framework. A unit represents part of the game-state and pef...
Definition: ISimulatedGameUnit.hh:28
This class should not be visible to developers at all.
Definition: SimulatedGameNode.h:25
Definition: UniqueTreeDataset.hh:23