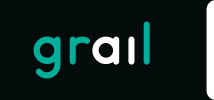 |
Grail (C++)
1.2.0
A multi-platform, modular, universal engine for embedding advanced AI in games.
|
3 #ifndef GRAIL_SIMULATED_GAME_UNIT
4 #define GRAIL_SIMULATED_GAME_UNIT
12 #include "ISimulatedGameAction.hh"
13 #include "ISimulatedGameHeuristic.hh"
19 using RandomGenerator = std::mt19937_64;
21 class SimulatedGameNode;
36 virtual void Reset() = 0;
47 virtual std::vector<std::unique_ptr<const ISimulatedGameAction>>
GetAvailableActions()
const = 0;
57 RandomGenerator& rand_gen)
const;
68 virtual std::string
ToString()
const;
85 virtual bool IsStochastic()
const = 0;
93 #endif //GRAIL_SIMULATED_GAME_UNIT
std::vector< std::unique_ptr< SimulatedGameHeuristic > > heuristicReasoners
Add or remove heuristic. See @SimulatedGameHeuristic. Heuristic is used to provide the action to play...
Definition: ISimulatedGameUnit.hh:79
virtual void FillDebugRepresentation(std::map< std::string, std::string > &nameValueDictionary) const
Method used to gather data for GUI-based debugging. Insert properties of the state (key,...
Definition: ISimulatedGameUnit.cpp:24
An interface that is used to terminate simulation in MCTS and set scores to players....
Definition: SimulatedGameRuntime.hh:17
virtual void AfterAction(SimulatedGameRuntime &)
This method is called after action.Apply(). You may insert custom logic here - for example - code tha...
Definition: ISimulatedGameUnit.cpp:15
The main interface class for the SimulatedGame reasoner based on the MCTS algorithm....
Definition: SimulatedGame.hh:30
virtual std::string ToString() const
Returns the text representation of the unit for debugging purposes. Default implementation returns an...
Definition: ISimulatedGameUnit.cpp:19
virtual std::unique_ptr< const ISimulatedGameAction > GetRandomAvailableAction(RandomGenerator &rand_gen) const
Returns a random action to be chosen in simulation. The default implementation samples from GetAvaila...
Definition: ISimulatedGameUnit.cpp:9
virtual int GetTeamIndex() const =0
Returns the index of a team. Units with the same team index share the same game score.
Base class of a unit in the SimulatedGame framework. A unit represents part of the game-state and pef...
Definition: ISimulatedGameUnit.hh:28
virtual void Reset()=0
Resets state of the unit to the beginning of the SimulatedGame.
virtual std::vector< std::unique_ptr< const ISimulatedGameAction > > GetAvailableActions() const =0
Returns the actions the unit may perform. It is strongly advised to create a new vector here,...
This class should not be visible to developers at all.
Definition: SimulatedGameNode.h:25