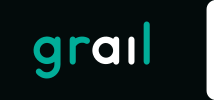 |
Grail (C++)
1.1.1
A multi-platform, modular, universal engine for embedding advanced AI in games.
|
4 #include "../Evaluator.hh"
5 #include "../../libs/vector2/vector2.h"
18 template <
typename ContextType>
27 : childEvaluator{childEvaluator}
31 Curve(
const Curve<ContextType>& other) =
default;
32 Curve(Curve<ContextType>&& other) =
default;
33 virtual ~
Curve() =
default;
40 virtual float Sample(
float argument)
const = 0;
45 return Sample(childEvaluator->EvaluateContext(context, snapshot));
49 virtual void DebugDump(
const std::map<const void*, std::size_t>& nodeMapping,
52 debugData.AddChildNode(nodeMapping.at(childEvaluator.get()));
56 std::shared_ptr<utility::Evaluator<ContextType>> childEvaluator{};
59 template <
typename ContextType>
73 :
Curve<ContextType>(childEvaluator), lowerBound{lowerBound}
77 virtual float Sample(
float argument)
const override final
79 return std::max(lowerBound, argument);
82 virtual EvaluatorType GetEvaluatorType() const override final {
return EvaluatorType::CURVE_LOWER_BOUND; }
85 float lowerBound = 0.0f;
88 template <
typename ContextType>
102 :
Curve<ContextType>(childEvaluator), upperBound{upperBound}
106 virtual float Sample(
float argument)
const override final
108 return std::min(upperBound, argument);
111 virtual EvaluatorType GetEvaluatorType() const override final {
return EvaluatorType::CURVE_UPPER_BOUND; }
114 float upperBound = 0.0f;
117 template <
typename ContextType>
134 :
Curve<ContextType>{childEvaluator}, lowerBound{lowerBound}, upperBound{upperBound}
138 virtual float Sample(
float argument)
const override final
140 return std::min(upperBound, std::max(lowerBound, argument));
143 virtual EvaluatorType GetEvaluatorType() const override final
145 return EvaluatorType::CURVE_DOUBLE_SIDED_BOUND;
149 float lowerBound = 0.0f;
150 float upperBound = 0.0f;
153 template <
typename ContextType>
160 std::vector<Vector2> DiscretizeCurve(
const Curve<ContextType>& curve, std::size_t samplesQuantity)
162 std::vector<Vector2> controlPoints;
163 float increment = 1.0f / (samplesQuantity - 1);
164 for(
float i = 0; i <= 1; i += increment)
166 controlPoints.push_back({i, curve.Sample(i)});
168 return controlPoints;
172 #endif //GRAIL_CURVE_H
DoubleSidedBound(std::shared_ptr< utility::Evaluator< ContextType >> childEvaluator, float lowerBound, float upperBound)
DoubleSidedBound - Constructor.
Definition: Curve.hh:131
The Curve class - Defines objects transforming one value into the other.
Definition: Curve.hh:19
virtual float Sample(float argument) const override final
Sample - Transforms argument into output value depending on the type of Curve.
Definition: Curve.hh:77
virtual float Sample(float argument) const override final
Sample - Transforms argument into output value depending on the type of Curve.
Definition: Curve.hh:106
virtual float Evaluate(const ContextType &context, UtilityEvaluatorSnapshot *const snapshot) const override final
Evaluate - Called from EvaluateContext which also evaluates context, but without automatically fillin...
Definition: Curve.hh:43
The UtilityEvaluatorSnapshot class - debug snapshot of whole evaluator tree assigned to evaluated obj...
Definition: UtilityEvaluatorSnapshot.h:21
virtual float Sample(float argument) const override final
Sample - Transforms argument into output value depending on the type of Curve.
Definition: Curve.hh:138
virtual void DebugDump(const std::map< const void *, std::size_t > &nodeMapping, EvaluationDebugData &debugData) const override final
DebugDump - Called from EvaluateContext, which generates additional debug data for each evaluator....
Definition: Curve.hh:49
The UpperBound class - Upper-bounds output of provided Evaluator.
Definition: Curve.hh:93
Curve(std::shared_ptr< utility::Evaluator< ContextType >> childEvaluator)
Curve - Constructor.
Definition: Curve.hh:26
The EvaluationDebugData class - debug data describing singular evaluator.
Definition: EvaluationDebugData.h:19
The LowerBound class - Lower-bounds output of provided Evaluator.
Definition: Curve.hh:64
The Evaluator class - base class being able to evaluate given context and output the result.
Definition: Evaluator.hh:20
The DoubleSidedBound class - Bounds output of provided Evaluator from both sides.
Definition: Curve.hh:122
UpperBound(std::shared_ptr< utility::Evaluator< ContextType >> childEvaluator, float upperBound)
UpperBound - Constructor.
Definition: Curve.hh:101
LowerBound(std::shared_ptr< utility::Evaluator< ContextType >> childEvaluator, float lowerBound)
LowerBound - Constructor.
Definition: Curve.hh:72
virtual float Sample(float argument) const =0
Sample - Transforms argument into output value depending on the type of Curve.